i3d::Image3d< VOXEL > Class Template Reference
The main template class which stores and manipulates the image data. More...
#include <image3d.h>
Inheritance diagram for i3d::Image3d< VOXEL >:
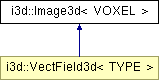
Public Member Functions | |
void | CopyFromVOI (const Image3d< VOXEL > &src, const VOI< size_t > &voi) |
function called by copy constructors | |
Image3d (const Offset *off_=0, const Resolution *res_=0) | |
Image3d (const char *fname, VOI< size_t > *voi=0, bool is_regex=false, int channel=-1, const Offset *off_=0, const Resolution *res_=0) | |
void | ReadImage (const char *fname, VOI< size_t > *voi=0, bool is_regex=false, int channel=-1, const Offset *off_=0, const Resolution *res_=0) |
void | SaveImage (const char *fname, FileFormat ft=IMG_UNKNOWN, bool comp=true) const |
Image3d (const Image3d &, const VOI< size_t > *voi=0) | |
Image3d (const Image3d &, const VOI< float > &voi) | |
virtual | ~Image3d () |
Image3d & | operator= (const Image3d &) |
VOXEL | GetVoxel (size_t x, size_t y, size_t z) const |
VOXEL | GetVoxel (const Vector3d< size_t > &v) const |
VOXEL | GetVoxel (size_t i) const |
void | SetVoxel (size_t i, VOXEL voxel) |
void | SetVoxel (size_t x, size_t y, size_t z, VOXEL voxel) |
void | SetVoxel (const Vector3d< size_t > &v, VOXEL voxel) |
VOXEL * | GetVoxelAddr (size_t x, size_t y, size_t z) |
const VOXEL * | GetVoxelAddr (size_t x, size_t y, size_t z) const |
VOXEL * | GetVoxelAddr (size_t i) |
const VOXEL * | GetVoxelAddr (size_t i) const |
VOXEL * | GetFirstVoxelAddr () |
VOXEL * | begin () |
VOXEL * | end () |
const VOXEL * | begin () const |
const VOXEL * | end () const |
const VOXEL * | GetFirstVoxelAddr () const |
bool | IsEmpty () const |
std::valarray< VOXEL > & | GetVoxelData () |
size_t | GetImageSize (void) const |
Get the number of voxels in image. | |
size_t | GetSliceSize (void) const |
Get the number of voxels per one slice. | |
size_t | GetWidth (void) const |
Get the width (horizontal axis) of the image. | |
size_t | GetHeight (void) const |
Get the height (vertical axis) of the image. | |
size_t | GetNumSlices (void) const |
Get number of slices per image. | |
size_t | GetSizeX (void) const |
Get the width (horizontal axis) of the image. | |
size_t | GetSizeY (void) const |
Get the height (vertical axis) of the image. | |
size_t | GetSizeZ (void) const |
Get number of slices per image. | |
size_t | GetX (size_t i) const |
Get the x-coordinate of the i-th voxel in the image data block. | |
size_t | GetY (size_t i) const |
Get the y-coordinate of the i-th voxel in the image data block. | |
size_t | GetZ (size_t i) const |
Get the z-coordinate of the i-th voxel in the image data block. | |
size_t | GetIndex (size_t x, size_t y, size_t z) const |
Coords | GetCoords (size_t i) const |
get the coords from the position in the image buffer vector | |
bool | OnBorder (const Vector3d< size_t > v) const |
Is (x,y,z) on the border of the image? | |
bool | Include (int x, int y, int z) const |
Is the voxel [x,y,z] in this image? | |
bool | Include (Vector3d< int > v) const |
Is the voxel 'v' in this image? | |
void | GetRange (VOXEL &min, VOXEL &max) |
Returns minimal and maximal value in the Image. Returns minimum and maximum in red green and blue, if the image is RGB or RGB16. | |
VOI< float > | GetVOI (void) const |
Get this image 'voi'. | |
Offset | GetOffset () const |
Get the offset of this image. | |
Resolution | GetResolution () const |
Get the resolution of this image. | |
Vector3d< size_t > | GetSize () const |
Get the dimensions of the image. | |
void | SetOffset (const Offset &off_) |
Set the offset for this image. | |
void | SetResolution (const Resolution &res_) |
Set the resolution for this image. | |
HistDesc | GetOrigHisto () const |
return original intensity histogram of the image | |
void | SetOrigHisto (const HistDesc &h) |
set new intensity histogram for current image | |
void | ComputeHistDesc (byte histo_smooth=std_histo_smooth, float thres_factor=std_thres_factor) |
void | DisposeData () |
void | MakeRoom (size_t width, size_t height, size_t slices) |
void | MakeRoom (const Vector3d< size_t > &sz) |
bool | TestConsistency (ImgVoxelType itype) const |
ImgVoxelType | ConsistentVoxelType () const |
Protected Attributes | |
Offset | offset |
position of the image in absolute coordinates in micrometers | |
Vector3d< size_t > | size |
size of the image (width x height x slices) in pixels | |
Resolution | resolution |
image resolution in pixels per micrometer | |
unsigned long | capacity |
size of memory allocated for data, in VOXELs | |
std::valarray< VOXEL > | data |
image data buffer | |
HistDesc | origHisto |
histogram of the original acquired image |
Detailed Description
template<class VOXEL>
class i3d::Image3d< VOXEL >
The main template class which stores and manipulates the image data.
Constructor & Destructor Documentation
i3d::Image3d< VOXEL >::Image3d | ( | const Offset * | off_ = 0 , |
|
const Resolution * | res_ = 0 | |||
) | [inline, explicit] |
Empty image:
i3d::Image3d< T >::Image3d | ( | const char * | fname, | |
VOI< size_t > * | voi = 0 , |
|||
bool | is_regex = false , |
|||
int | channel = -1 , |
|||
const Offset * | off_ = 0 , |
|||
const Resolution * | res_ = 0 | |||
) |
Read image from a specified file and constructs the Image3d object
parameters:
- voi ... if you want to read only specific rectangular par of the image
- 'is_regex' says, whether the input 'fname' is regular filename or can be expected as a mask for regular expansion.
- 'channel' chooses the channel order which is going to be read. If default value (-1) is chosen, all the channels are read. For the time being, only ICS format supports this parameter.
- offset and resolution set the image layout in the real scene. Both of them are optional: if not set, the values stored in the image data file is used (implicitly), otherwise the argument values are used (explicitly).
i3d::Image3d< VOXEL >::Image3d | ( | const Image3d< VOXEL > & | , | |
const VOI< size_t > * | voi = 0 | |||
) |
i3d::Image3d< VOXEL >::Image3d | ( | const Image3d< VOXEL > & | , | |
const VOI< float > & | voi | |||
) |
virtual i3d::Image3d< VOXEL >::~Image3d | ( | ) | [inline, virtual] |
Destructor:
Member Function Documentation
void i3d::Image3d< T >::ReadImage | ( | const char * | fname, | |
VOI< size_t > * | voi = 0 , |
|||
bool | is_regex = false , |
|||
int | channel = -1 , |
|||
const Offset * | off_ = 0 , |
|||
const Resolution * | res_ = 0 | |||
) |
Reads 3D image from specified file. Returns true on success.
parameters:
- voi ... if you want to read only specific rectangular par of the image
- 'is_regex' says, whether the input 'fname' is regular filename or can be expected as a mask for regular expansion.
- 'channel' chooses the channel order which is going to be read. If default value (-1) is chosen, all the channels are read. For the time being, only ICS format supports this parameter.
- offset and resolution set the image layout in the real scene. Both of them are optional: if not set, the values stored in the image data file is used (implicitly), otherwise the argument values are used (explicitly).
open image
read the basic image info to the predefined structures
verify if the required VOI is included in the image
fetch the image size
choose the channel to read if the default value is set (-1), then all the channels are read
test if image file is in the same colorspace as the prepared image structure
set some image characteristics, only if requested:
set the image offset (if set)
test whether the resolution is defined by the user
test whether the resolution is defined within the image format
allocate the memory
read the image data
void i3d::Image3d< T >::SaveImage | ( | const char * | fname, | |
FileFormat | ft = IMG_UNKNOWN , |
|||
bool | comp = true | |||
) | const |
SaveImage: Saves the image into a file(s) in specified format File format is guessed from extension of fname if ft == IMG_UNKNOWN
IOException is thrown
- if format for saving is not recognized or not supported
- if some disk problems occurred
create image writer
set the image dimensions
set the image voxel type (binary, gray8, rgb, ...)
set the image resolution (if it is even used)
set the referred image compression
set the image offset
save header info
save the data
Image3d& i3d::Image3d< VOXEL >::operator= | ( | const Image3d< VOXEL > & | ) |
Asignment operator :
VOXEL i3d::Image3d< VOXEL >::GetVoxel | ( | size_t | x, | |
size_t | y, | |||
size_t | z | |||
) | const [inline] |
Get a voxel value (voxels are numbered from 0):
VOXEL i3d::Image3d< VOXEL >::GetVoxel | ( | const Vector3d< size_t > & | v | ) | const [inline] |
Get a voxel value (voxels are numbered from 0)
VOXEL i3d::Image3d< VOXEL >::GetVoxel | ( | size_t | i | ) | const [inline] |
Get a voxel value (voxels are numbered from 0)
void i3d::Image3d< VOXEL >::SetVoxel | ( | size_t | i, | |
VOXEL | voxel | |||
) | [inline] |
Set a voxel to value (voxels are numbered from 0)
void i3d::Image3d< VOXEL >::SetVoxel | ( | size_t | x, | |
size_t | y, | |||
size_t | z, | |||
VOXEL | voxel | |||
) | [inline] |
Set a voxel to value (voxels are numbered from 0)
void i3d::Image3d< VOXEL >::SetVoxel | ( | const Vector3d< size_t > & | v, | |
VOXEL | voxel | |||
) | [inline] |
Set a voxel to value (voxels are numbered from 0)
VOXEL* i3d::Image3d< VOXEL >::GetVoxelAddr | ( | size_t | x, | |
size_t | y, | |||
size_t | z | |||
) | [inline] |
Get a voxel address (voxels are numbered from 0)
const VOXEL* i3d::Image3d< VOXEL >::GetVoxelAddr | ( | size_t | x, | |
size_t | y, | |||
size_t | z | |||
) | const [inline] |
Get a voxel address (voxels are numbered from 0)
VOXEL* i3d::Image3d< VOXEL >::GetVoxelAddr | ( | size_t | i | ) | [inline] |
Get a voxel address (voxels are numbered from 0)
const VOXEL* i3d::Image3d< VOXEL >::GetVoxelAddr | ( | size_t | i | ) | const [inline] |
Get a voxel address (voxels are numbered from 0)
VOXEL* i3d::Image3d< VOXEL >::GetFirstVoxelAddr | ( | ) | [inline] |
Get the first voxel address (voxels are numbered from 0)
VOXEL* i3d::Image3d< VOXEL >::begin | ( | ) | [inline] |
Synonym for GetFirstVoxelAddr()
VOXEL* i3d::Image3d< VOXEL >::end | ( | ) | [inline] |
Pointer beyond the last image element
const VOXEL* i3d::Image3d< VOXEL >::begin | ( | ) | const [inline] |
Synonym for const GetFirstVoxelAddr()
const VOXEL* i3d::Image3d< VOXEL >::end | ( | ) | const [inline] |
Const pointer beyond the last image element
const VOXEL* i3d::Image3d< VOXEL >::GetFirstVoxelAddr | ( | ) | const [inline] |
Get the first voxel address (voxels are numbered from 0)
bool i3d::Image3d< VOXEL >::IsEmpty | ( | ) | const [inline] |
Is the image empty?
std::valarray<VOXEL>& i3d::Image3d< VOXEL >::GetVoxelData | ( | ) | [inline] |
Returns the reference the the object 'data', which is of the std::valarray
data type
size_t i3d::Image3d< VOXEL >::GetIndex | ( | size_t | x, | |
size_t | y, | |||
size_t | z | |||
) | const [inline] |
Get the position of the voxel in the image data block (first position is O.
void i3d::Image3d< T >::ComputeHistDesc | ( | byte | histo_smooth = std_histo_smooth , |
|
float | thres_factor = std_thres_factor | |||
) |
Compute histogram and its descriptionn for this image and store them to origHisto. Voxel lying at the border are not considered.
void i3d::Image3d< VOXEL >::DisposeData | ( | ) | [inline] |
Free memory occupied by data and set size to (0,0,0)
void i3d::Image3d< VOXEL >::MakeRoom | ( | size_t | width, | |
size_t | height, | |||
size_t | slices | |||
) | [inline] |
Allocate/reallocate voxel memory and set the image size. The input are three dimensions.
void i3d::Image3d< VOXEL >::MakeRoom | ( | const Vector3d< size_t > & | sz | ) | [inline] |
Allocate/reallocate voxel memory and set the image size. The input is the dimension vector.
bool i3d::Image3d< T >::TestConsistency | ( | ImgVoxelType | itype | ) | const |
Test, whether Image3d<T> can, in principle, be created from a file of type itype:
ImgVoxelType i3d::Image3d< T >::ConsistentVoxelType | ( | ) | const |
Return a file type consistent with the voxel type T:
The documentation for this class was generated from the following files:
- image3d.h
- image3d.cc